Android RecyclerView in Kotlin
RecyclerView is a UI (User Interface) component in Android development that provides a flexible and efficient way to display large data sets in a scrollable list or grid format. It is an improved version of the older ListView and GridView components, offering better performance and more advanced features.
The primary purpose of RecyclerView is to efficiently manage the creation and recycling of personal item views as they appear and disappear from the screen. In addition, this recycling mechanism allows RecyclerView to handle large datasets without consuming excessive memory or causing performance issues.
RecyclerView works based on the concept of an adapter. Therefore, you need to create an adapter class that extends the RecyclerView.Adapter, which binds the data to individual views within the RecyclerView. The adapter is responsible for creating the views, securing data, and handling user interactions.
The RecyclerView also uses a LayoutManager to arrange the items in the list or grid format. Different types of LayoutManagers are available, such as LinearLayoutManager, GridLayoutManager, and StaggeredGridLayoutManager, each providing different ways to display the items.
The RecyclerView offers several benefits, including:
- Efficient memory usage: The recycling mechanism decreases the views requiring creation, resulting in improved memory management.
- Smooth scrolling: RecyclerView provides smooth scrolling, even with large datasets, by reusing the existing views instead of creating new ones.
- Customizability: It allows you to customize the appearance and behavior of individual items by creating custom item layouts and view holders.
- Animation support: RecyclerView supports animations when items are added, removed, or changed, providing a visually appealing user experience.
- Item interactions: It simplifies handling user interactions, such as clicks or swipes, on individual items by providing built-in event listeners.
In this tutorial, we will learn how to use RecyclerView, write a small example, and see how to use RecyclerView in Kotlin Activity. Here is a RecyclerView android example.
To start, add the dependency to your app in the build.gradle file: implementation ‘androidx.recyclerview:recyclerview:1.2.1’
Now create a .xml layout file with info about your item.
<!-- item_layout.xml --> <TextView android:id="@+id/tvItem" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="16dp" android:textSize="18sp" />
In it, we will create TextView, and define its Id, width, height, padding, and text size.
After this, create RecyclerView.Adapter class and implement onCreateViewHolder, onBindViewHolder, and getItemCount methods to your code
class MyAdapter: RecyclerView.Adapter < MyAdapter.ViewHolder > () { var items = listOf < String > () class ViewHolder(view: View): RecyclerView.ViewHolder(view) { val item: TextView = view.findViewById(R.id.tvItem) } override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder { val view = LayoutInflater.from(parent.context).inflate(R.layout.item_layout, parent, false) return ViewHolder(view) } override fun onBindViewHolder(holder: ViewHolder, position: Int) { val item = items[position] holder.item.text = item } override fun getItemCount() = items.size }
We need onCreateViewHolder to create a new ViewHolder, onBindViewHolder to connect data to it, getItemCount, and get the overall number of items in our dataset.
Methods in the RecyclerView.Adapter class
Let’s dive into more detail about the different methods in the RecyclerView.Adapter class.
- onCreateViewHolder: This method is responsible for creating a new ViewHolder instance. The RecyclerView calls it when it needs a new ViewHolder to represent an item in the list.
- In the onCreateViewHolder method, you inflate the layout for the item view using the LayoutInflater from the provided parent ViewGroup. You pass the layout resource ID (in this case, R.layout.item_layout) to the inflate method along with the parent ViewGroup and false to indicate that you don’t want to attach the inflated view to the parent immediately.
- Once you have the inflated view, you create a new instance of the ViewHolder class, passing the view as a parameter and returning it.
- onBindViewHolder: The RecyclerView calls this method to bind the data to the ViewHolder. It is invoked for each item in the dataset as the user scrolls through the list.
- In the onBindViewHolder method, you retrieve the data item at the specified position from the items list (which you should set before calling setAdapter on the RecyclerView).
- Then, you use the ViewHolder parameter to access the views within the item layout. In this case, you set the text of the TextView with the retrieved data item.
- getItemCount: This method returns the total number of items in the dataset. The RecyclerView must know how many things are present to determine the list’s size and enable proper scrolling behavior.
- In the getItemCount method, you return the size of the items list.
These three methods work together to create and bind the data to the views within the RecyclerView in Kotlin. By overriding them in the MyAdapter class, you customize the behavior to fit your specific data and item layout.
Remember to set the MyAdapter instance as the adapter for your RecyclerView using recyclerView.adapter = MyAdapter(), and also select the items list with the appropriate data before setting the adapter.
That’s it! With these methods implemented, you can efficiently display and manage a list of items using RecyclerView in Kotlin.
Now let’s create a .xml file for RecyclerView, which will contain its height and width:
<!-- activity_main.xml --> <androidx.recyclerview.widget.RecyclerView android:id="@+id/rvItems" android:layout_width="match_parent" android:layout_height="match_parent" />
The developer creates a separate “item_layout.xml” file to define the layout for each item in the RecyclerView. In addition, it specifies the UI components and their properties that make up each item’s appearance.
So, we have everything we need for our RecyclerView, except we only use it in a place.
class MainActivity: AppCompatActivity() { private lateinit var listAdapter: MyAdapter override fun onCreate(savedInstanceState: Bundle ? ) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) //first, we create an adapter setupRecyclerView() //then we dynamically change its content according to our needs listAdapter.items = getListOfItems() } private fun setupRecyclerView() { val rvItems = findViewById < RecyclerView > (R.id.rvItems) with(rvItems) { listAdapter = MyAdapter() layoutManager = LinearLayoutManager(this @MainActivity) adapter = listAdapter } } private fun getListOfItems(): List < String > = listOf("Item 1", "Item 2", "Item 3", "Item 4", "Item 5") }
Now you should have a working list with your items. You can customize it, change from list to grid, add your items or animation, etc.
Passing data from the RecyclerView adapter to an Activity
To display information about your item, filter a dataset, or update it, you sometimes must pass data from RecyclerView to an Activity.
To do so, add a lambda function to your RecyclerView adapter. It should look like this:
var onClickListener: ((String) -> Unit)? = null
In this example, we will handle all the clicks to MyAdapter class.
Now, let’s rewrite our onBindViewHolder class in the RecyclerView adapter, and add lambda property to pass our information to the Activity
class MyAdapter: RecyclerView.Adapter < MyAdapter.ViewHolder > () { var items = listOf < String > () var onClickListener: ((String) - > Unit) ? = null // ... override fun onBindViewHolder(holder: ViewHolder, position: Int) { val item = items[position] holder.item.text = item holder.itemView.setOnClickListener { onClickListener ? .invoke(item) } } // ... }
In the onBindViewHolder method, we set an onClickListener on the itemView (root view of the item layout). When users click the itemView, it triggers the onClickListener and invokes the associated lambda function with the corresponding item data.
Now, let’s modify our MainActivity to handle the click events:
class MainActivity: AppCompatActivity() { private lateinit var listAdapter: MyAdapter override fun onCreate(savedInstanceState: Bundle ? ) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) setupRecyclerView() listAdapter.items = getListOfItems() listAdapter.onClickListener = { item - > // Handle the click event here Toast.makeText(this, "Clicked item: $item", Toast.LENGTH_SHORT).show() } } private fun setupRecyclerView() { val rvItems = findViewById < RecyclerView > (R.id.rvItems) with(rvItems) { listAdapter = MyAdapter() layoutManager = LinearLayoutManager(this @MainActivity) adapter = listAdapter } } }
private fun getListOfItems(): List<String> = listOf(“Item 1”, “Item 2”, “Item 3”, “Item 4”, “Item 5”)
In this example, we set the onClickListener property of the listAdapter in MainActivity to a lambda function that shows a toast message with the clicked item’s data.
Passing data from the RecyclerView adapter to an Activity allows you to perform various actions based on user interactions with the items in the RecyclerView. In the previous example, we demonstrated how to handle click events on individual items and pass the clicked item’s data to the Activity using a lambda function.
FAQ
Can I put RecyclerView in an activity?
Yes, you can put a RecyclerView in an activity. RecyclerView is a UI component that users can use in activities and fragments. You can create a RecyclerView in an activity similar to an activity by adding the RecyclerView to the activity’s layout file and setting up the RecyclerView in the activity’s code.
How can I put RecyclerView in an activity?
To put a RecyclerView in an activity, you need to follow these steps:
1. Add the RecyclerView to the activity’s layout file (XML) by defining its width, height, and other properties.
2. Find the RecyclerView using its ID in the activity’s code and store it in a variable.
How to use RecyclerView using Kotlin?
Create a View element in the xml file, find the stuff by its id in the activity, and save it in a variable. Create an adapter class. Initialize the adapter in the activity and install the recycler-created adapter. After that, you can send data in the specified format to the recycler through the adapter and receive callbacks when interacting with the elements in the list.
How to pass data from RecyclerView to an activity?
To pass data from a RecyclerView to an activity, you can use an interface, a callback, or a lambda mechanism. Lamda is the general approach. By implementing this pattern, you can pass data from the RecyclerView adapter to the containing Activity or Fragment, handle the click events, or perform other desired actions based on the clicked item’s data.
Recommends
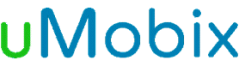
One App to manage all your Agile/
Scrum/Devops Needs
All your work in one place: Task, Project Managment, Bug Tracker, IT Assets, CRM, Docs, Excel, Chat, Goals and more.
Get Free