Enum Classes
Enum classes are a vital feature in Kotlin that allows you to determine a kit of constants, also noted as enums, in a type-safe manner. In this article, we’ll look at the concept of Kotlin enum class, how to utilize them, and some helpful techniques that go with them.
Kotlin Enum Classes: Introduction
Enum classes are determined utilizing the “enum class” keyword, according to the enum name of the class. For illustration:
enum class Fruit { APPLE, BANANA, KIWI }
In this code snippet, we have determined an enum class named “Fruit” with four enum constants: APPLE, BANANA, and KIWI. Every constant represents a distinct value that the enum class can take.
Anonymous classes
Enum constants can announce their anonymous classes with techniques and override basic ways.
enum class DownloadState { PREPARING { override fun state() = DOWNLOADING }, DOWNLOADING { override fun state() = PREPARING }; abstract fun state(): DownloadState }
If the enum class determines any members, utilize a semicolon to single the constant and member declarations.
Implementing interfaces in enum classes
Enum classes can implement interfaces such as regular classes. Enum classes are like Java enum types but offer more flexibility and power.
Here’s an enum illustration of implementing an interface in an enum class:
interface TypeOfFruit { fun printType() } enum class Fruit: TypeOfFruit { APPLE { override fun printType() { println("Apple") } }, BANANA { override fun printType() { println("Banana") } }, KIWI { override fun printType() { println("Kiwi") } } }
In this illustration, we determine a TypeOfFruit interface with a single technique printType(). Then we announce a Kotlin enum class named Fruit and make it implement the TypeOfFruit interface. Every enum constant in the Fruit enum overrides the printType() technique with its implementation.
We may utilize this enum class as follows:
fun main() { Fruit.APPLE.printType() // Output: Apple Fruit.BANANA.printType() // Output: Banana Fruit.KIWI.printType() // Output: Kiwi }
When invoking the printType() technique on the enum constant, it executes the overridden implementation specific to that constant.
Enum classes are strong and may have characteristics, techniques, and custom behavior for every constant. As a result, they offer much flexibility and can be an excellent choice for representing a stable kit of values with associated behaviors.
Working with Enum Constants
An enum is a particular type that represents a group of related constants. Enum constants are determined utilizing the enum keyword and may have characteristics, techniques, and associated values. Operating with enum constants allows you to choose a stable kit of values and act on them.
enum class Fruit { APPLE, BANANA, KIWI }
The code snippet determines an enum named Fruit with four constants: APPLE, BANANA, and KIWI. A comma singles out every constant.
Now, let’s see how you could utilize these enum constants:
fun main() { val fruit = Fruit.APPLE // Accessing enum constants println(fruit) // Output: APPLE // Comparing enum constants if (fruit == Fruit.BANANA) { println("It is a banana") } else { println("It isn’t a banana") } // Enum iteration val allFruits = Fruit.values() for (enumValue in allFruits) { println(enumValue) } }
In the code above, we make a variable fruit and assign it the value Fruit.APPLE. We may approach enum constants utilizing the dot notation (Fruit.APPLE). We can also compare enum constants using the == operator.
To iterate over all the enum constants, we utilize the values() function, which returns an array of all the enum constants. We can then loop over the array to print every enum constant.
Enums may have characteristics and techniques as well. Here’s an illustration:
enum class Fruit(val amount: Int) { APPLE(10), BANANA(10), KIWI(20); fun printAmount() { println("Amount: $amount") } }
We added characteristic amounts to every enum constant in the updated Fruit enum. We also added a technique printAmount(), that prints the quantities associated with every constant.
Users could approach the characteristic and call the technique on an enum constant:
fun main() { val fruit = Fruit.APPLE println(fruit.amount) // Output: 10 fruit.printAmount() // Output: Amount: 10 }
That’s the basic idea of working with enum constants. You may determine custom enum constants with associated characteristics and techniques and utilize them as a stable kit of meanings in your code.
Receiving Enum Constants by Name
To get an enum constant by its String name, we use enumValueOf() static function:
enum class Fruit { APPLE, BANANA, KIWI } fun main() { val fruit: Fruit = enumValueOf("APPLE") println(fruit) // Output: APPLE }
FAQ
How to get an enum based on the value in Kotlin?
To receive an enum based on a specific value, you may utilize the enumValueOf() function or the valueOf() function. For illustration, you may use Fruit if you have an enum named Fruit and wish to retrieve the enum constant based on the enum with the value “APPLE” .valueOf(“APPLE”) or enumValueOf(“APPLE”). Both functions will return the enum constant that matches the given meaning.
Can enums have values?
Yes, enums in Kotlin may have values associated with every constant. You may assign characteristics or additional data to every enum constant. In addition, enum constants may have their unique kit of features, like regular classes. These meanings can be accessed and utilized within the enum class or when working with enum illustrations.
Can I set a value to an enum?
Yes, you may set values to an enum. Enum constants may have characteristics assigned to them, allowing you to store specific meanings or data for every constant. These users can determine implications directly in the constant enum declaration or class body. You may approach and modify these meanings as needed in your code.
How do you give a value to an enum?
To give a value to an enum constant in Kotlin, you may determine characteristics directly in the constant enum declaration or the enum class body. For example, here’s a Kotlin enum illustration:
enum class Fruit(val amount:Int) {
APPLE(10),
BANANA(10),
KIWI(20)
}
In this illustration, the Fruit enum has three constants (APPLE, BANANA, and KIWI), and every continuous has an associated meaning characteristic of type Int. You may assign any meaning or data type you demand to the features of your enum constants.
Please note that distinct meanings must be provided when defining the enum constants and can’t be changed afterward, as enum constants are immutable.
Recommends
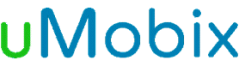
One App to manage all your Agile/
Scrum/Devops Needs
All your work in one place: Task, Project Managment, Bug Tracker, IT Assets, CRM, Docs, Excel, Chat, Goals and more.
Get Free