How to Create a Splash Screen in Android?
A Splash Screen on Android adds value to an app as it is the first impression for users. Creating a bespoke splash screen for your Android can set the tone of the whole user experience to come, and this article will show how to do so.
What is a splash screen on Android?
It is the graphical element that materializes a logo or any visual content while an app loads.
In the world of mobile apps and games, one effective strategy for sharing important content or branding efforts with users is by implementing splash screens.
How to make a splash screen on Android?
Customizing an Android app’s splash screen takes some effort, but here’s how you can get started:
1. Open up Android Studio and begin working on a brand new project while providing an appropriate name and setting the minimum API levels required, or you also can add it to an existing project.
2. Begin by generating a layout resource file using the “New” option in the “res” folder before channeling your creativity into designing a one-of-a-kind introduction that includes images.
3. To display the splash screen when opening an application, you need to modify its manifest file. In particular, you need to make changes to the main activity section of this document. This involves adding this piece of code:
<activity android:name=".StartActivity" android:theme="@style/SplashTheme" android:screenOrientation="portrait"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity>
Executing this programming sequence specifies “SplashTheme” as your app’s theme and dictates portrait mode as the preferred orientation. It also sets the display of the splash screen when opening the application.
4. You can customize the splash screen by setting its background. For example, you can use any color (also a gradient) or logo. To do this, you need to create a drawable file, for example, “splash_background”. And in our example, we’re adding a gradient as the background for the splash screen:
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle"> <gradient android:angle="135" android:endColor="#B1AFEA" android:startColor="#E8D2D6" android:type="linear" /> </shape>
“startColor” and “endColor” – these colors can be any.
Here you can see how it will be displayed:
5. To configure a splash screen that stands out, add the following code to your styles.xml file and create a unique style.
<style name="SplashTheme" parent="Theme.AppCompat.Light.NoActionBar"> <item name="android:background">@drawable/splash_background</item> <item name="colorPrimary">@color/colorPrimary</item> <item name="colorPrimaryDark">@color/colorPrimaryDark</item> <item name="colorAccent">@color/colorAccent</item> <item name="android:statusBarColor">@color/colorAccent</item> </style>
Your new style will be named “SplashTheme” and it inherits from the parent theme “Theme.AppCompat.Light.NoActionBar.” Using this code, you can customize your splash screen by setting its background using the drawable called “splash_background”.
6. Now StartActivity is running.
StartActivity is the launch screen from which the program starts its work. In this activity(StartActivity) you can also check some conditions, for example, whether the user is logged into the application or not. On this screen, we determine which screen should be opened first. And in this example, it is enough to run MainActivity without any preconditions.
To do this, add the following code to your StartActivity.kt file:
class StartActivity: AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle ? ) { super.onCreate(savedInstanceState) startActivity(Intent(this @StartActivity, MainActivity::class.java)) finish() } }
In this activity, we don’t need to use the setContentView() method because we already set the SplashTheme in the manifest, and the system takes that theme to display on the screen at startup.
7. Before you finish developing your application, you should continue testing to ensure that your newly implemented splash screen displays properly. It should be displayed for a short period before revealing the main activity of your application. But if you have a high-performance phone, you might not see it.
Image size in the drawable folder
In most cases, app_logo.png should always be placed in the drawable-xxhdpi folder, as the logo will automatically resize to fit all types of cell phone screens.
Also, make sure that the resolution of the image does not exceed 1000×1000 pixels. Depending on the resolution selected, it may be less than that. There are currently a number of splash screen styles with numerous logos placed around the perimeter of the screen. Is such a thing possible?
Simply put as many items from the layer list as you need on the splash screen.
<layer-list xmlns:android="http://schemas.android.com/apk/res/android"> <!-- Add as many items as needed --> <item> <bitmap android:src="@drawable/app_logo" android:gravity="center" /> </item> <!-- Add more items here if desired --> </layer-list>
In the above code, we use a layer-list drawable to define multiple items for the splash screen. The <item> tag is used to specify each individual item within the layer-list. In this case, we include a <bitmap> item to display the app_logo.png image at the center of the screen using android:gravity=”center”.
Splash Screen API
With the release of Android 12, a new splash screen API has been implemented. With Android 12, all apps now display a splash screen by default, with the app’s symbol in the center. Android 12’s default splash screen first, which sounds great until you realize that if you are using an activity or fragment to display a splash screen, you will then see your own splash screen. Now you have two splash screens, and they are likely to be very different from each other. More Unified Design and User Penetration We need to add support for Android 12 to give the splash screen a more unified look and feel, and to help it penetrate the user.
This API provides backward compatibility with previous API levels, along with support for animated icons via AnimatedVectorDrawable or AnimationDrawable. Have a cup of coffee and continue working on this project. Once complete, you will have an animated splash screen that matches the theme of your device.
About the Settings
There are a few settings that need to be done in your app before you can access the new API. We will take advantage of the backward compatibility of this API and include the splash screen in previous Android releases.
1. In the app module build.gradle, modify the compileSdk; set Gradle’s Splash Screen API dependency to version 31. For backward compatibility, use the compact version.
android { compileSdkVersion 31 // Other configurations... } dependencies { implementation 'androidx.appcompat:appcompat:1.4.0' implementation 'com.google.android.material:material:1.5.0' // Add the Splash Screen API dependency implementation 'androidx.core:core-splashscreen:1.0.0-alpha01' }
2. Create two documents with the same name splash theme.xml. Both should be placed in the values directory, one in values-night. Create two files, values and values-night, to match the dark or light mode theme of the user’s device. Give both files the same name to make them easier to organize for future updates.
3. In both files, create a splash screen theme for the dark and light mode scenarios where Theme.SplashThemeName is the name of the theme and is derived from Theme.SplashScreen. The file should now look like this:
styles.xml (for Dark mode):
<resources> <!-- Other styles --> <style name="Theme.SplashThemeName" parent="Theme.SplashScreen"> <!-- Customize the theme for Dark mode → <item name=”windowSplashScreenBackground">@color/dark_splash_background</item> <item name="windowSplashScreenAnimatedIcon">@mipmap/ic_my_launcher</item> <item name="windowSplashScreenAnimationDuration">200</item> <item name="postSplashScreenTheme">@style/Theme.MaintTheme</item> <!-- Status bar and Nav bar configs --> <item name="android:statusBarColor" tools:targetApi="l">@color/dark_status_bar</item> <item name="android:navigationBarColor">@color/dark_nav_bar</item> <item name="android:windowLightStatusBar">false</item> </style> </resources>
styles.xml (for Light mode):
<resources> <!-- Other styles --> <style name="Theme.SplashThemeName" parent="Theme.SplashScreen"> <!-- Customize the theme for Light mode → <item name="android:windowSplashScreenBackground”>@color/light_splash_background</item> <item name="windowSplashScreenAnimatedIcon">@mipmap/ic_my_launcher</item> <item name="windowSplashScreenAnimationDuration">200</item> <item name="postSplashScreenTheme">@style/Theme.MaintTheme</item> <!-- Status bar and Nav bar configs --> <item name="android:statusBarColor" tools:targetApi="l">@color/light_status_bar</item> <item name="android:navigationBarColor">@color/light_nav_bar</item> <item name="android:windowLightStatusBar">true</item> </style> </resources>
In the above code snippets, we assume that you have separate colors defined for the dark and light splash screen backgrounds, `@color/dark_splash_background` and `@color/light_splash_background` respectively. Replace them with the actual color resources you have defined in your project.
Detailing the contents
— The background color of the splash screen is controlled by `windowSplashScreenBackground`.
— The splash screen icon, `windowSplashScreenAnimatedIcon`, should have circular borders and be centered. It should be designed to work with earlier API levels and should not exceed 108dp in size to prevent clipping.
— The `windowSplashScreenAnimationDuration` determines how long the animated icon is displayed. It doesn’t affect the animation itself but can be useful for customizing the exit animation.
— After the splash screen, you can specify the app’s actual theme using `postSplashScreenTheme`.
— To ensure a consistent appearance, set the status bar and nav bar attributes in the splash screen theme to match the `windowSplashScreenBackground`.
— Customize the settings for the splash screen theme’s dark and light modes to achieve the desired appearance.
By considering these points, you can effectively configure and customize your splash screen theme in Android.
4. Set the android:theme attribute of the application tag to the Splash Screen theme you created earlier by opening the manifest file. They are style:Theme.SplashThemeName.
<manifest xmlns:android=”http://schemas.android.com/apk/res/android” package=”com.yourpackage.name”> <application android:theme=”@style/Theme.SplashThemeName” ...> ... </application> </manifest>
5. InstallSplashScreen() must be used in the entrance activity (the activity that enters from the launcher), immediately after super.onCreate() and before setContentView() in the onCreate method. See this documentation:
class MainActivity: AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle ? ) { super.onCreate(savedInstanceState) // Install the splash screen InstallSplashScreen() // Set the activity layout setContentView(R.layout.activity_main) // Rest of your code... } // Rest of your activity code... }
Well, that’s it — we have successfully included Splash not only in Android 12, but also in earlier Android releases.
This icon will be clipped if it is larger than 108dp. Icons can be drawable, png, jpg, or webp. animatedVectorDrawable or AnimationDrawable will only work with Android 12 and not with lower API levels, so it is now a win can’t be added to dowSplashScreenBackground. Animation icons are only available in Android version 12 and above. If set to all API levels, you will see a blank field for lower API levels. That is not what we want. Further customization can be done via extra visual elements like animations and sound. Including various display resolutions or adding a progress bar are ways to tailor splash screens depending on the screen or device being used, letting users know when the app is loading.
Android Studio’s layout editor or custom animations through the Android Animation API can be implemented for visual elements within this opening feature.
Different splash screens can be created for different screen sizes or resolutions by utilizing Android’s resource qualifier system along with multiple layout files to specify different resources corresponding to varying screen densities. A progress bar indicating loading times or customized loading indicators using animations and other visual elements can be added to enhance the user experience of the splash screen.
Check out the related video tutorial on how to create a splash screen in Android Studio using Kotlin.
FAQ
What is the difference between a launch screen and a splash screen in Android?
The launch screen and splash screen are different screens in Android, which are used when an Android application launches.
The splash screen, also known as the “app icon screen,” is the screen that appears when the user taps on the app icon. It is a static image that only appears for a brief moment and disappears when the app finishes loading.
The launch screen is a mandatory component of the app and is required by the Android platform that appears after the splash screen and before the main screen.
Therefore, the main difference between the splash screen and the launch screen is that the splash screen appears immediately after the app is launched, while the launch screen appears after the splash screen during app loading.
How to make a splash screen on Android?
- Design your splash screen layout: Create a layout XML file (`splash_screen.xml`) defining the UI elements for your splash screen, such as an image or logo.
- Create a new activity: Create a new activity (`SplashActivity.kt`) that will serve as your splash screen.
- Set the splash screen as the launcher activity: In your AndroidManifest.xml file, specify the splash activity as the launcher activity by adding the `intent-filter` with the `MAIN` and `LAUNCHER` actions.
- Set a theme for the splash activity: In your styles.xml file, define a theme (e.g., `Theme.Splash`) for the splash activity, specifying a full-screen layout and any other desired visual properties.
- Implement a delay or background operation: In the `onCreate` method of your splash activity, you can add a delay using `Handler` or perform any necessary background operations.
- Start the next activity: After the delay or completion of background operations, start the next activity using an intent, typically the main activity of your app.
- Test your splash screen: Run your app to verify that the splash screen is displayed for the specified duration and transitions to the main activity as intended.
What is a splash screen on Android?
A splash screen on Android is a graphical element that is displayed when an app is launched. It typically appears for a brief period, providing a visual indication to the user that the app is loading or initializing.
Recommends
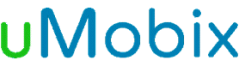
One App to manage all your Agile/
Scrum/Devops Needs
All your work in one place: Task, Project Managment, Bug Tracker, IT Assets, CRM, Docs, Excel, Chat, Goals and more.
Get Free