How to get the class in Kotlin? – getClass()
If you’re a Kotlin developer, you may have encountered instances when you needed to access the class facility of a specific sample. In Java, you typically use the .getClass() method to obtain the class object. However, Kotlin provides a more concise and intuitive way to achieve a similar result. This article will explore different approaches to getting the class in Kotlin and understand the alternative of .getClass().
Using ::class to get the class object
You can utilize the ::class syntax to obtain the class facility of a given sample. For example, let’s consider a piece where we have a class called Fruit:
class Fruit(val name: String, val amount: Int) val apple = Fruit("Apple", 30) val fruitClass = fruit::class
Here, fruit::class returns the class facility presenting the Fruit class. It’s a concise and straightforward way to retrieve the class in Kotlin.
In Kotlin, you can use reflection to access the properties of a class after obtaining its class object with the::class syntax. The examination allows you to observe and modify classes, attributes, and methods while they are running.
Here’s an example of how you can get the properties of the Fruit class and access their values:
import kotlin.reflect.full.declaredMemberProperties class Fruit(val name: String, val amount: Int) val fruit = Fruit("Apple", 30) val fruitClass = fruit::class // Get the declared properties of the class val properties = fruitClass.declaredMemberProperties // Iterate over the properties and access their values for (property in properties) { val propertyName = property.name val propertyValue = property.get(fruit) println("$propertyName: $propertyValue") }
In this example, declaredMemberProperties is a property of the KClass class, which returns a list of properties declared in the class. We iterate over these properties and use the get function to retrieve their values from the person object.
Output:
- name: Apple
- amount: 30
Using reflection at runtime, you can dynamically access and manipulate properties, functions, and other class members. However, it’s important to note that consideration can impact performance.
Getting the class name
To retrieve the class name as a string, you can use the .simpleName property of the class facility. Continuing with the previous sample, we can obtain the class name below:
val fruitClassName = fruit::class.simpleName
In this case, the value “Fruit” will be assigned to fruitClassName. ::class.simpleName can be helpful when you dynamically obtain the class name for logging or other purposes.
Retrieving the class object using javaClass
The javaClass property returns the class facility and works similarly to the .getClass() method in Java.
The difference between ::class and ::class.java
The difference between ::class and ::class.java lies in the type of class object they return and the reflection capabilities they provide:
- ::class: When utilized on a class reference or an object instance, ::class returns the KClass instance representing the class. The KClass is a Kotlin-specific class that provides reflection capabilities specific to Kotlin. With KClass, you can access properties and functions defined in the class, check its modifiers (e.g., isAbstract, isOpen, isSealed), retrieve type parameters, and perform other Kotlin-specific operations. It allows you to work with Kotlin-specific features and extensions.
Example:
val fruitClass: KClass<FruitClass> = FruitClass::class
- ::class.java: When utilized on a class reference or an object instance, ::class.java returns the java.lang.Class instance representing the class. It is the Java class object for the Kotlin class. It provides reflection capabilities based on the Java programming language. With Class, you can access properties and functions defined in the class, check its modifiers (e.g., isInterface, isEnum, isAnnotation), retrieve annotations, work with generic types using Java’s type system, and perform other Java-specific operations. It allows you to leverage Java-based reflection capabilities.
Example:
val fruitClass: Class<FruitClass> = FruitClass::class.java
So ::class returns a KClass instance with Kotlin-specific reflection capabilities, while ::class.java returns a java.lang.Class instance with reflection capabilities based on the Java programming language. The choice between them depends on whether you need to work with Kotlin-specific or Java-based reflection features.
And there is also such a method “::class.java.”
The difference between ::class.java and .javaClass
In Kotlin, there is a difference between ::class.java and .javaClass when accessing the Java Class object of a Kotlin class:
People utilize the .javaClass expression to retrieve the Java Class object representing the runtime class of a Kotlin instance. For example, users employ it when there is an instance of a class and they wish to access its class object dynamically.
On the other hand, developers employ the ::class.java syntax to access the Java Class object of a Kotlin class itself. It returns the Class object that represents the Kotlin class. Developers commonly use this approach when they need to access the class object statically without requiring an instance of the class.
Therefore, the primary distinction lies in their usage. For example, the Kotlin class itself employs `::class.java` to access its class object, whereas an instance of a Kotlin class uses `.javaClass` to access its class object.
Summary table
To summarize the different approaches discussed:
Approach | Call Code | Explanation |
---|---|---|
1 | ::class | Utilize the ::class syntax to obtain the class object. It returns a KClass instance representing the class with Kotlin-specific reflection capabilities. You can access properties and functions defined in the class, check modifiers, retrieve type parameters, and perform other Kotlin-specific operations. |
2 | ::class.simpleName | Use the ::class.simpleName property to retrieve the class name as a string. This can be useful for dynamic logging or other purposes. |
3 | .javaClass | People use this syntax to obtain the class reference as a Java Class object. It’s similar to ::class but provides interoperability with Java code. You can use it to access Java-specific reflection features or when there is an instance of a class and you wish to access its class object dynamically. |
4 | ::class.java | When you append .java to ::class, you obtain the Java Class object of the class. ::class is similar to .javaClass and is useful for interoperability with Java. It allows you to use Java-specific reflection methods and APIs on the class reference. You can use it when they need to access the class object statically without requiring an instance of the class. |
FAQ
How do I get a class type in Kotlin?
In Kotlin, you can get the class type in multiple ways:
1. Using the ::class syntax:
val fruitClassType1 = FruitClass::class
2. Using the ::class.java syntax:
val fruitClassType2 = FruitClass::class.java
3. Using the .javaClass property:
val objFruitClass = FruitClass()
val fruitClassType3 = objFruitClass.javaClass
All three approaches will give you the class type of FruitClass.
What does getClass() do?
The getClass() method is utilized in Java to obtain the class facility of a particular sample. However, in Kotlin, the alternative way to achieve a similar result is using the ::class syntax. For sample, if you have a sample obj of a class MyFruitClass, you can use fruitObj::class to get the class facility.
What is the equivalent of .class in Kotlin?
In Kotlin, the alternative of the .class syntax in Java is ::class. This syntax allows you to obtain the class facility of a given sample. For sample, if you have a sample obj of a class MyFruitClass, you can use fruitObj::class to get the class facility.
How to get a class name in Kotlin Android?
To get the class name in Kotlin Android, you can use the::class.simpleName property. This property returns the name of the class as a string. For sample, if you have a class MyFruitClass, you can get its name by using MyFruitClass::class.simpleName.
Recommends
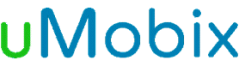
One App to manage all your Agile/
Scrum/Devops Needs
All your work in one place: Task, Project Managment, Bug Tracker, IT Assets, CRM, Docs, Excel, Chat, Goals and more.
Get Free