Initializing by lazy and lateinit variables in Kotlin
In Kotlin, the lateinit and by lazy keywords ensure convenient ways to handle parameter beginning. They ensure flexibility and efficiency when working with variables that are not always available or are expensive to calculate. This article explores the concepts of lateinit and by lazy in Kotlin, their differences, and when to use each.
lateinit keyword
The lateinit keyword allows us to declare non-nullable variables without immediately initializing them. These variables must be of type var and their beginning is deferred until later in code execution. However, using a lateinit parameter before beginning results in a NullPointerException.
by lazy keyword
The by lazy function is a delegated property that calculates its value only the 1st time it is approached. It takes a lambda expression that is evaluated and cached, and then approaching the parameter returns the cached value. By default, by lazy properties are immutable (val) and thread-safe.
Features of lateinit work
Expanding on my last point, lateinit can be used well with non-primitive data types. It can only be applied to non-primitive types, such as classes and objects. The functionality is not compatible with primitive types such as long or int. This limitation arises because whenever a lateinit property is approached, Kotlin internally assigns it a null value to signify that the property has not yet been initialized.
When working with lateinit variables in Kotlin, it is crucial to ensure that they are properly begun before approaching them. Failing to initialize a lateinit parameter before usage will result in a NullPointerException. Here are some approaches for handling uninitialized lateinit variables:
Initializing in the same scope: Initialize the lateinit parameter within the same function or scope before any usage of the variable. This ensures that the parameter is begun before it is approached.
lateinit var testVar: String fun setupVar() { testVar = "Test value" //Set value for variable 'testVar' }
Using a null check: Perform a null check before approaching the lateinit parameter to ensure it has begun. This helps avoid the risk of NullPointerException. Using the isInitialized property: Kotlin ensures the isInitialized property to check if a lateinit parameter has been begun. You can use this property to conditionally handle the beginning status of the variable.
fun checkIsVarInitialized() { if (::testVar.isInitialized) { // Use testVar safely println(testVar) } else { // Handle the case when testVar is not initialized } }
Features of by lazy work
Through the delegate method, lazy beginning is accomplished using the by lazy keyword. The lambda expression you want to use to run the code to initialize the property is what the lazy delegate accepts.
When a parameter needs to be declared, beginning is sometimes postponed. Initialization delays can have a wide range of causes. For instance, beginning necessitates pre-computation, which is preferable to avoid if the outcome won’t ever be used. In these circumstances, the parameter is typically declared as var, which initially has the value null and remains that way forever.
var phoneModel: String ? = null ... phoneModel = getPhoneModel() //assigning a value fun getPhoneModel(): String { return“ some phone model” }
This is problematic if you wish to prevent null values and know the parameter will have a specific value. While it may be of type String in our case, the parameter is of type String?, which permits null. Alternatively, you might use a predetermined value, like in:
var phoneModel: String = "NOTHING" // or the empty string var phoneModel: String = "" ... phoneModel = getPhoneModel()
However, even though the variable’s value won’t change after beginning, you still need to utilize var. Kotlin advises using by lazy in certain circumstances:
val phoneModel: String by lazy { getPhoneModel() }
In this example, the getPhoneModel() function will be called only once, when phoneModel is retrieved for the 1st time. You can also use a function reference instead of a lambda expression:
val phoneModel: String by lazy(::getPhoneModel)
Differences between lateinit and by lazy
The main differences between lateinit and by lazy in Kotlin are as follows:
- Initialization time:
- lateinit: lateinit variables are initialized before their 1st usage, typically within the same function or scope. The beginning can occur at a later point in the code execution, but approaching a lateinit parameter before beginning will result in a NullPointerException.
- by lazy: by lazy properties are initialized when they are 1st approached. The beginning is deferred until the property is approached for the 1st time, which may happen at a different point in the program execution.
- Mutability:
- lateinit: lateinit variables are mutable (var), meaning their value can be reassigned after beginning. They can be used when the value needs to be updated or changed after beginning.
- by lazy: by lazy properties are immutable (val), and their value cannot be changed once initialized. They are useful for scenarios where you don’t want the value to change after it’s 1st calculated.
- Nullability:
- lateinit: lateinit variables must be of a non-nullable type and cannot be assigned a null value. If a lateinit parameter is approached before being initialized, a NullPointerException will be thrown.
- by lazy: by lazy properties can be assigned null values if their type allows it. However, once initialized, the value is fixed and cannot be null.
- Usage Scenarios:
- lateinit: lateinit is useful when you have a non-nullable property that cannot be initialized immediately but will be assigned a value before usage. It is commonly used in dependency injection scenarios or when properties are initialized from external sources.
- by lazy: by lazy is beneficial when you want to defer the computation or beginning of a property until it is 1st approached. It is suitable for by lazy beginning, memoization, or situations where resource allocation is expensive and should be delayed until necessary.
It is essential to consider these differences when choosing between lateinit and by lazy based on the specific requirements and constraints of your Kotlin code.
Conclusion
In Kotlin, the lateinit and by lazy keywords offer powerful tools for parameter beginning. While lateinit is suitable for scenarios where the value will be assigned before usage, by lazy ensures deferred computation and efficient resource allocation. Understanding the differences between these two approaches allows developers to make informed decisions on which option to use based on the specific requirements of their code. By leveraging lateinit and by lazy, developers can improve performance, handle complex beginning scenarios, and optimize resource allocation.
To further enhance your understanding of lateinit and by lazy in Kotlin, I recommend watching the following video: lateinit vs by lazy in Kotlin.
Additionally, here is a code example showcasing the usage of lateinit and by lazy:
class ExampleClass { lateinit var lateInitVar: String val a = 37 val b = 5 val lazyVal: Int by lazy { // Perform expensive computation or beginning here a + b } fun setupVar(newValue: String) { lateInitVar = newValue } } //now we turn to these variables fun main() { val testClass = TestClass() // approaching lateInitVar with initialization testClass.setupVar("New value") println(testClass.lateInitVar) // Prints "New value" println(testClass.lazyVal) // Performs computation and prints 42 println(testClass.lazyVal) // Returns cached value of 42 }
In this example, the lateInitVariable is declared using lateinit and is initialized by calling the setupVar()’ function. Attempting to approach lateInitVar before beginning would result in a NullPointerException.
On the other hand, the by lazyVal is a by lazy property that performs an expensive computation when approached for the 1st time. Subsequent approaches to by lazyVal return the cached value without recomputation.
In conclusion, understanding the differences between lateinit and by lazy in Kotlin enables developers to handle parameter beginning efficiently and effectively. By leveraging these keywords appropriately, developers can improve the performance and maintainability of their Kotlin codebase.
FAQ
What is a lateinit in Kotlin?
In Kotlin, the lateinit keyword allows us to declare non-nullable variables without initializing them immediately. These variables are initialized at a later point before their 1st usage, but approaching a lateinit parameter before beginning results in a NullPointerException.
Why not use lateinit in Kotlin?
While lateinit ensures flexibility for parameter beginning, it also introduces the risk of NullPointerException if the parameter is approached before being initialized. It should only be used if there is a guarantee that the parameter will be initialized before use.
What is the difference between by lazy and lateinit in Kotlin?
The main differences between by lazy and lateinit in Kotlin are as beginning time, mutability, and nullability.
What is the alternative to lateinit in Kotlin?
In Kotlin, the alternative to using lateinit is to declare a property as nullable and initialize it with a default value. This ensures that the property is always initialized before being approached.
Here’s an example:
class TestClass {
var test: String? = null
}
In this example, test is declared nullable (String?) and is initialized with a default value of null. If you try to approach myProperty before assigning a value to it, it will be null. You can then assign a value to it later in your code.
This approach ensures that you don’t encounter a NullPointerException when approaching the property before it’s initialized, but it does require handling the possibility of the property being null when using it.
Why not use by lazy in Kotlin?
While by lazy ensures efficiency by lazy beginning, it may not be suitable for scenarios where the property’s value needs to be mutable (var).
What is by lazy initialization in Kotlin?
By lazy beginning in Kotlin refers to the practice of deferring the computation or beginning of a property until it is 1st approached. The value is calculated only once and then cached, ensuring that subsequent approaches return the cached value without recomputation.
When should I use lateinit in Kotlin?
Lateinit can be used in situations where a non-nullable property needs to be initialized at a later point, such as when the value is ensured from external sources or through dependency injection. It can also be used to improve performance by avoiding immediate initialization of properties that are costly to compute.
Remember to refer to the article for more in-depth explanations and examples regarding the usage of lateinit and by lazy in Kotlin.
Recommends
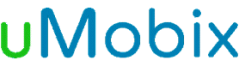
One App to manage all your Agile/
Scrum/Devops Needs
All your work in one place: Task, Project Managment, Bug Tracker, IT Assets, CRM, Docs, Excel, Chat, Goals and more.
Get Free