Kotlin Arrays – Everything You Need to Know
In this blog post, we’ll cover everything you need to know about functional data objects and everything from introducing what an array is to the details of how Kotlin handles arrays. Ultimately, you’ll have a comprehensive understanding of how to loop through elements and modify their values.
Creating Array in Kotlin
Creating an array in Kotlin requires using the arrayOf()(arrayOf<T>() ) function or Array() constructor. Here are some examples:
Using the arrayOf() function
// creates an array of integers val numbers = arrayOf(1, 2, 3, 4, 5) // creates an array of strings val fruits = arrayOf("apple", "banana", "orange")
In those examples, we use the arrayOf() function to create an array of integers and an array of strings. The elements of the array are specified in a comma-separated list within the parentheses.
Using the Array() constructor
// creates an array of integers with a size of 5 val numbers = Array(5) { index -> index * 2 } // creates an array of strings with a size of 3 val fruits = Array(3) { index -> "fruit ${index + 1}" }
In those examples, we use the Array() constructor to create an array of integers and an array of strings. The first argument to the constructor specifies the size of the array, and the second argument is a lambda function that defines the values of the elements. In the lambda function, the index parameter is the index of the current element being initialized.
How to Use an Array() Constructor
Kotlin provides two ways to create an array, one is through the use of arrayOf(), arrayOf<T> and Array().
Array(size: Int, init: (Int) -> T) – this constructor creates an array of the specified size, where each element is initialized using the lambda expression init, which takes an index as its parameter and returns the value for that element.
val numberArray = Array(5) { i -> i * 2 } // Creates an array of size 5 with elements [0, 2, 4, 6, 8]
We can also create an array without an initializer:
val stringArray = Array(3) { "" }
This creates a new array of strings with size 3, where each element is initialized to an empty string.
Arrays in Kotlin are mutable by default, which means we can modify their elements after they have been created. For example, we can change the value of an element at a specific index as follows:
stringArray[0] = "First"
This code changes the value of the first element in the intArray from “” to “First”.
Overall, the Array() structure provides a convenient and powerful way to work with collections of elements in Kotlin.
Creating array using arrayOf() and arrayOf<T>() functions
Creating an array of a particular type in Kotlin can be done using the arrayOf() and arrayOf<Int> functions.
The arrayOf() function takes in a vararg of items as its argument and creates an array out of it. For example, if you want to create a collection of integers, you can do so by calling:
val intArray = arrayOf(1, 2, 3, 4, 5)
The arrayOf<Int> function takes in two arguments – the type of the elements and the number of elements. The syntax for this is:
val intArray2 = arrayOf<Int>(10)
This will create an array of 10 elements with default values. In this case, all the elements are 0. You can also specify different values as parameters.
Also, we can create an array of mixed data types using arrayOf() like so:
val mixedArray = arrayOf("Kotlin", 3, 5.7, true) println("Mixed Array: ${mixedArray.joinToString()}") Similarly, you can create an array of integers using arrayOf<Int>() like this: val intArray = arrayOf<Int>(1, 2, 3, 4, 5) println("Integer Array: ${intArray.joinToString()}")
It’s important to note that both functions are effective when you know the number of elements at compile-time.
Alternatively, you can use them to create an empty array. For example, you can create an empty string array like this:
val emptyArray = arrayOf<String>() println("Empty Array: ${emptyArray.joinToString()}")
This creates an empty String array that is ready to be filled with values. An alternative method of creating an empty array with the same result would look like this:
val myArray = emptyArray<String>()
Primitive type arrays
Kotlin also provides an array for primitive types like Int, Double, Float, Long, Byte, Char, Short, Boolean. They provide the same benefits as standard arrays but use less memory. Therefore, they allow you to work with large amounts of data more efficiently. To create a primitive type, you must use the arrayOfPrimitives() function, as shown in the example below:
val intArray = intArrayOf(1,2,3)
This creates an int array of length 3, with each element being the number indicated. Primitive type arrays can also be declared with values already filled in, such as in the following example:
kotlin val intArray = intArrayOf(1, 2, 3) val longArray = longArrayOf(4L, 5L, 6L) val floatArray = floatArrayOf(1.1f, 2.2f, 3.3f) val doubleArray = doubleArrayOf(1.1, 2.2, 3.3) val byteArray = byteArrayOf(0x21, 0x55, 0x5c) val shortArray = shortArrayOf(1, 2, 3) val booleanArray = booleanArrayOf(true, false) val charArray = charArrayOf('a', 'b', 'c')
Each line creates a new array of the specified type and initializes it with the values in the parentheses. The intArrayOf, longArrayOf, floatArrayOf, doubleArrayOf, byteArrayOf, shortArrayOf, booleanArrayOf, and charArrayOf functions create functions with specific data types.
Also, you can use the PrimitiveArray() function, as shown in the example below:
val intArray = IntArray(6) intArray[0] = 11 intArray[1] = 22 intArray[2] = 33 intArray[3] = 44 intArray[4] = 55 intArray[5] = 66
This created an int array with the values 11, 22, 33, 43, 55, and 66 in that order.
Note that you can’t add or remove elements from an array once it’s created. If you need to store more or less than 6 values, you would need to create a new array with the appropriate size.
Accessing Elements in an Array
Kotlin’s array type allows for easy access to its elements. The syntax is pretty straightforward and similar to Java. To access a specific element in an array, you use the bracket notation with the index number as the argument. For example:
val items = arrayOf("apple", "banana", "orange") println(items[2]) // prints "orange"
If you want to access the third element (in this case “orange”), then you specify the index of 2. Keep in mind that the first element has an index of 0. Besides, if you try to access an element outside of the bounds, an exception will be thrown.
Also, you can use the second way, to get access to an element from the array by the get() function.
println(items.get(2)]) // prints "orange"
The get() method is functionally equivalent to using square bracket notation. This method gets the element by index, too.
The third way, used first and last properties: You can get the first and last elements of an array using the first and last properties, respectively. For example:
println(items.first()) //print apple println(items.last()) //print orange
The fourth way is – ElementAt() method. You can use the elementAt() method to get an element at a specific index. This method takes an index as a parameter and returns the element at that index, or throws an exception if the index is out of bounds. For example:
println(items.elementAt(2)) //print orange
The elementAt() method is the same as using the square bracket notation or the get() method, but with the added safety of throwing an exception if the index is out of bounds.
mutableListOf vs. arrayOf
mutableListOf and arrayOf are two Kotlin classes used to define collections of elements. A mutableListOf is an interface that supports both read and write operations, while an arrayOf is a class that can hold a fixed number of elements. When it comes to choosing between the two, there are pros and cons to each class:
mutableListOf | arrayOf |
---|---|
Allows for easy adding, removing, and modifying of elements | Offers better performance for read-only lists and arrays |
Can be used for both mutable and read-only lists | Supports primitive types through specialized functions |
Provides useful extension functions for common operations | Provides useful extension functions for common operations |
Type inference allows for more concise code | Can have more compact syntax for small read-only lists |
Can have performance issues with very large lists | Cannot add, remove, or modify elements after initialization |
Requires explicit type declaration for non-generic lists | Not as flexible as mutableListOf |
Depending on the size of the list and the type of operations you will be performing on it, one might be better than the other. If you need more flexibility, use mutableListOf, but if you need faster access times and fewer memory issues, use arrayOf.
How to call mutableListOf and arrayOf
mutableListOf: It is a function that creates a new MutableList, which is a part of the Kotlin Collections Framework. A MutableList is a dynamic array with flexible size can add, remove, or update elements during runtime.
val mutableList = mutableListOf(1, 2, 3, 4) mutableList.add(5) // Adds the item 5 at the end of the MutableList println(mutableList)
Output: [1, 2, 3, 4, 5]
arrayOf: It is a function that creates an Array, which is a built-in collection type. An Array is a fixed-size collection, which means its size cannot be changed. You can update the elements in an Array but cannot add or remove elements.
val array = arrayOf(1, 2, 3, 4) array[2] = 5 // Updates the 3rd element to the value 5 println(array.toList())
Output: [1, 2, 5, 4]
Converting Between mutableListOf and arrayOf
Typically, Kotlin allows you to use both the mutableListOf and arrayOf functions to create arrays. Moreover, you can use them interchangeably. To convert between the two, you need to use the .toMutableList() or .toTypedArray() functions.
How to convert between MutableList and Array in Kotlin
Convert MutableList to Array using the toTypedArray() method:
val mutableList = mutableListOf(1, 2, 3, 4) val array = mutableList.toTypedArray()
Convert Array to MutableList using the toMutableList() method
val array = arrayOf(1, 2, 3, 4) val mutableList = array.toMutableList()
Here’s an example with different data types:
// for string val mutableListString = mutableListOf("A", "B", "C", "D") val arrayString = mutableListString.toTypedArray() val arrayString = arrayOf("A", "B", "C", "D") val mutableListString = arrayString.toMutableList()
How to Loop Through an Array
Looping through an array is one of the most important and useful operations that you can perform in Kotlin. It allows you to iterate through each item in the array and do something with it. There are several different ways to loop through an array in Kotlin:
Using a for loop
val array = arrayOf(1, 2, 3, 4, 5) for (element in array) { println(element) }
Using the forEach method
val array = arrayOf(1, 2, 3, 4, 5) array.forEach { element -> println(element) }
Using the forEachIndexed method (useful when you also need the index)
val array = arrayOf(1, 2, 3, 4, 5) array.forEachIndexed { index, element -> println("Index: $index, Element: $element") }
No matter which looping option you choose, it’s important to remember to check the bounds of your array before attempting to loop through it. This will prevent any IndexOutOfBounds exceptions from occurring.
Final Thoughts
Kotlin Arrays are a powerful and versatile tool to store data in an organized way. They are fast and efficient and allow for quick lookups and traversal through elements. With the help of arrayOf() and Array() functions, you can easily create arrays in Kotlin. Ultimately, you can iterate through them using the loop structure and primitive type arrays.
FAQ
What are arrays in Kotlin?
Arrays in Kotlin are objects that store multiple values of the same or mixed type. They provide a convenient way to store and access data and can be used to loop through elements and modify their values.
How do I create a Kotlin array?
You can create an array in Kotlin using the arrayOf() or arrayOf<Int> function. You can also use the Array() constructor to create an array from an existing collection.
What is the type of array in Kotlin?
In Kotlin, the type of array is Array<T>, where T represents the type of elements stored in the array. For example, Kotlin provides specialized array classes for primitive types like IntArray, CharArray, DoubleArray, and others to avoid the overhead of boxing/unboxing.
How to read an array in Kotlin?
You can loop through an array and access each element using its index – nameArray[index]. Alternatively, you can use the get() function to access a specific element by its index.
Recommends
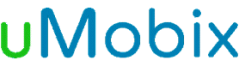
One App to manage all your Agile/
Scrum/Devops Needs
All your work in one place: Task, Project Managment, Bug Tracker, IT Assets, CRM, Docs, Excel, Chat, Goals and more.
Get Free