Kotlin Extension Function: How to Create and Use Them
Extension functions in Kotlin allow you to add more functionality without changing the source code of existing classes. Let’s go over the basics and see how to create and use extension functions in your project.
What is an Extension Function in Kotlin?
Thanks to the availability of extension functions, we can add supplementary features to present classes without changing their initial source code. This capability is particularly useful when working with unmodifiable or externally controlled classes where additional functionalities would otherwise remain inaccessible. Kotlin facilitates the creation of new extensions for both built-in and user-defined classes enhancing modularity while creating a more expressive coding environment. The resultant improved implementation supports functional enrichment through better class performance within a given application context.
Extension functions in Kotlin provide several benefits, including:
- Enhanced code reuse ability: Extension functions allow you to reuse code in your project as many times as needed without duplicating it in multiple places.
- Improved reuse ability: Extension functions can make your code better readable and concise by encapsulating logic to reusable functions.
- Simpler syntax: Extension functions can simplify the syntax of your code by providing a natural and intuitive way to call functions.
How to create an extension function in Kotlin
To create an extension function in Kotlin, you need:
- Define a function outside the class you want to extend.
- Prefix the name of the function with the class name you want to extend.
- Add the `.` operator before calling the extension function.
- Specify the function’s parameters and return type as you would in a regular function.
For instance, an extension function that adds a `reverse()` function to the String class:
fun String.reverse(): String { return this.reversed() }
Therefore, we define an extension function named `reverse()` in the String class. This will reverse the characters in the string using the function `reversed()` provided by Kotlin.
The defined extension function can function as if it were part of the class being extended, just like a member function. Here is an example of using the `reverse()` extension function we just created:
val originalString = "Hello, World!" val reversedString = originalString.reverse() println(reversedString) // Output: !dlroW ,olleH
Here, the `reverse()` function is called on a string object (`originalString`) as if it were a built-in function. The result (the reversed string) is stored in the `reversedString` variable and output to the console.
Another example is replacing the first letter of a string with a capital letter using the Kotlin extension function:
fun String.capitalizeFirstLetter(): String { if (this.isEmpty()) { return "" } return this.substring(0, 1).toUpperCase() + this.substring(1) }
Extension functions can be incorporated into the code base to extend the functionality of the `String` class. This particular function, known as `capitalizeFirstLetter()` works by capitalizing only the first character within a defined string and then providing us with its updated version.
Another Kotlin extension function example of creating may be an extension for a custom class:
class Car(val make: String, val model: String, val year: Int) fun Car.printDetails() { println("Make: $make, Model: $model, Year: $year") }
Here we have created an extension function called `printDetails()` which extends the `Car` class. This function prints the make, model, and year of manufacture of the vehicle.
When it comes to improving existing class traits with ease and elegance, nothing beats using Kotlin extension functions! These valuable elements have proven incredibly useful due to their flexible nature – not only do they allow for greater code reuse but also result in better readability throughout coding sequences while simultaneously facilitating modular design practices within larger project contexts. Using these extensions will take your development experience up a notch regarding efficiency and enjoyment.
Also, watch the Kotlin Extension Function Tutorial video for better memorization of information.
FAQ
What is an example of an extension function in Kotlin?
A sample of an extension function in Kotlin is adding the `capitalizeFirstLetter()` function to the `String` class, which capitalizes the first letter of a string:
fun String.capitalizeFirstLetter(): String {
if (this.isEmpty()) {
return “”
}
return this.substring(0, 1).toUpperCase() + this.substring(1)
}
How do you call a Kotlin extension function?
To execute a Kotlin extension function, there is no need for any elaborate steps. Simply apply it to your targeted object that demands expansion, using the `.` operator. To illustrate this point, let’s look at an example: `myString.capitalizeFirstLetter()`, where “capitalizeFirstLetter()” is the extension function.
Where do I put Kotlin extension functions?
These functions may be located anywhere within the project’s source code. It is recommended to save them in a separate file for orderliness of the code.
What is the benefit of the extension function in Kotlin?
The advantage is that developers can add functionality to existing classes without having to inherit from them or change the source code. The result is cleaner, more concise code that is also more readable and maintainable.
How do extension functions work in Kotlin?
Kotlin’s extension functions allow new functions to be added without modifying the source code of existing classes. They provide a way to extend the functionality of a class, even standard library classes or classes from external libraries, without the need for inheritance or wrapping.
Recommends
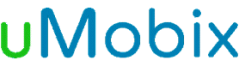
One App to manage all your Agile/
Scrum/Devops Needs
All your work in one place: Task, Project Managment, Bug Tracker, IT Assets, CRM, Docs, Excel, Chat, Goals and more.
Get Free