Kotlin listOf() – How to create list
In case you are working on an application using Kotlin programming language, it might be necessary for you to handle lists. Kotlin offers various alternatives for generating and managing lists, and among them is the ‘listOf()’ function.
This post will cover the usage of the ‘listOf()’ function for generating lists, getting elements from lists, displaying lists, and other related operations.
Using ‘listOf()’ in Kotlin
To form a list that can’t be altered later, the ‘listOf()’ method is implemented in Kotlin. Here’s a sample code illustrating the creation of a list of integers with the use of ‘listOf'():
val numbers = listOf(1, 2, 3, 4, 5)
You can also use the listOf<T>() function to create a list, where T can be any primitive data type in Kotlin. In Kotlin, the primitive data types include Int, Double, Float, Long, Short, Byte, Char, Boolean, and their corresponding nullable versions (e.g., Int?, Double?, etc.). Generating a list of strings with the ‘listOf<String>()’ function in the following code:
val fruits = listOf<String>("apple", "banana", "orange")
To append elements to an existing list, the ‘plus’ operator can be employed, as shown in the code below:
val numbers = listOf(1, 2, 3) val moreNumbers = numbers + 4 + 5
The resulting list ‘moreNumbers’ will be [1, 2, 3, 4, 5]. The ‘plus’ operator returns a new list that contains all the elements of the original list ‘numbers’ and the new elements ‘4’ and ‘5’. Since lists in Kotlin are immutable, the original list ‘numbers’ remains unchanged.
Accessing elements in a list
To access an element in a list, you can use the index operator ‘[]’ or use the ‘get()’ function with the element’s index:
val fruits = listOf("apple", "banana", "orange")
An example using the index operator ‘[]’:
val secondFruit = fruits[1] // "banana"
An example using the ‘get()’ function with the element’s index:
val firstFruit = fruits.get(0) // "apple"
Printing a list
To print a list, you can use a for loop or the ‘forEach’ function:
val fruits = listOf("apple", "banana", "orange") for (fruit in fruits) { println(fruit) } // OR fruits.forEach { println(it) }
The output of both the for loop and the forEach function in this code will be: apple, banana, orange
Kotlin listOf() Functions
Function | Description |
---|---|
‘get’ | Returns the element at the specified index |
‘indexOf’ | Returns the index of the first occurrence of the specified element in the list, or -1 if the element is not found |
‘contains’ | Returns true if the list contains the specified element |
‘subList’ | Returns a new list containing the elements between the specified start and end indices |
‘size’ | Returns the number of elements in the list |
Here’s an example of how to create a list of ‘Person’ objects and print their names:
data class Person(val name: String, val age: Int) fun main() { val people = listOf( Person("John", 25), Person("Jane", 30), Person("Bob", 20) ) for (person in people) { println(person.name) } }
The code will output the following: John, Jane, Bob
It creates a list of ‘Person’ objects, containing the name and age of each person. Then it uses a for loop to iterate over the list and print the name of each person.
Final Thoughts
To summarize, ‘listOf()’ function provides a convenient means to create an immutable list in Kotlin. This feature enables accessing elements of the list with the index operator or with the get() function. For adding and removing items, we can use mutable lists in Kotlin which have their own set of functions and methods. Additionally, you can use ‘for’ loops or the ‘forEach’ function to print the elements of a list.
We hope this article has provided you with a better understanding of how to work with lists in Kotlin. For more information on collections in Kotlin, check out the related video on Kotlin Collections Tutorial – Understanding List, Set & Map. With the help of code examples, screenshots, and tables, we have tried to cover all the essential aspects of Kotlin lists.
FAQ
What is 'listOf()' in Kotlin?
‘listOf()’ is a built-in function in Kotlin that is used to create an immutable list. It takes zero or more elements as its arguments and returns an instance of List, which is an interface representing an ordered collection of elements.
How do I make a list of strings in Kotlin?
An instance of a string list can be produced in Kotlin with the aid of the ‘listOf()’ function.
For instance, ‘val fruits = listOf(“apple”, “banana”, “orange”)’.
How do I get an element from a list in Kotlin?
The index operator ‘[]’ or the ‘get()’ function in Kotlin can be utilized to obtain an element from a list.
val fruits = listOf(“apple”, “banana”, “orange”)
val secondFruit = fruits[1] // “banana”
val firstFruit = fruits.get(0) // “apple”
Recommends
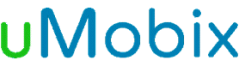
One App to manage all your Agile/
Scrum/Devops Needs
All your work in one place: Task, Project Managment, Bug Tracker, IT Assets, CRM, Docs, Excel, Chat, Goals and more.
Get Free