Kotlin Sealed Class: Exploring Powerful Abstractions
The kotlin sealed class is a unique and forced construct that lets creators designate bounded class pecking orders with a bounded bunch of subclasses. It brings additional control and flexibility to the class-based paradigm, enabling the creation of strong and expressive code. We’ll go through the concept of sealed classes in detail, including their objective, use, and privileges.
Concertation on Sealed Classes
1. The Kotlin Sealed Class Overview:
- Utilizing the sealed modifier defines a sealed class, which restricts the class’s descent to finite subclasses designated within the same file.
- People commonly utilize sealed classes to present bounded kinds, such as an original bunch of info states or alternative outcomes of an operation.
2. Privileges of Sealed Classes:
- Enhanced kind Security: Sealed classes help eliminate runtime mistakes by defining a closed bunch of subclasses, ensuring comprehensive handling of all feasible cases.
- Enhanced Abstraction: Sealed classes let a concise way to present complex state-based logic, promoting clean and self-contained code.
- Template Matching: Kotlin’s sealed classes make it less difficult to employ template matching, enabling creators to promote numerous subclasses and run particular code blocks based on the sealed class instance.
3. Diversities Between Sealed Class and Other Modifiers:
- Final vs sealed: While last bounds up further subclassing, sealed lets a bounded count of subclasses. Viewers could perceive sealed classes as a fixed version of abstract classes.
- Internal vs sealed: The internal modifier regulates the visibility of a declaration within a module, while the sealed modifier controls the ranking and restricts the count of possible subclasses for a sealed class.
Utilizing Sealed Classes
See a scenario of modeling kinds of literature. It is the subclass of the head-sealed class ‘Literature.’ ‘Scientific’ and ‘Fiction’ are subclasses inherited from ‘Literature’ and override the ‘getNumberOfPages’ method.
sealed class Literature { abstract fun getNumberOfPages() } class Scientific: Literature() { override fun getNumberOfPages() { println("Scientific contains 200 pages") } } class Fiction: Literature() { override fun getNumberOfPages() { println("Fiction contains 100 pages") } } fun getPageByTypeOfBook(literature: Literature) { when(literature) { is Fiction - > literature.getNumberOfPages() is Scientific - > literature.getNumberOfPages() } } fun main() { val scientific = Scientific() val fiction = Fiction() getPageByTypeOfBook(scientific) // Output: Scientific contains 200 pages getPageByTypeOfBook(fiction) // Output: Fiction contains 100 pages }
Sealed Interfaces in Kotlin
The kotlin sealed class restricts access similarly to a sealed interface. It differs from a sealed class in that it lacks state and behavior. The system uses sealed interfaces to establish a constrained type range for interface implementation. You must declare all sealed interface implementations in the same file as the sealed interface.
sealed interface Literature { class Fiction(val page: Int): Literature class Scientific(val page: Int, val color: String): Literature }
FAQ
What is a sealed class in Kotlin?
A sealed class in Kotlin is a unique class declaration that bounds the class’s descent to a finite digit of subclasses appointed inwardly of the item file. Sealed classes present related class pecking orders for modeling a closed bunch of related info states or alternative outcomes.
What is a sealed class?
Generally speaking, a sealed class is a concept found in programming languages, inclusively Kotlin. It presents a class that bounds the descent to a finite count of subclasses. Sealed classes are applied, designated closed, and managed to peck orders of related classes.
What is the difference between the final and sealed class in Kotlin?
While final restricts future subclassing, sealed allows for unlimited subclasses. You can think of sealed classes as a bounded version of abstract classes.
What is the difference between the internal and sealed class in Kotlin?
The internal modifier controls the visibility of a declaration within a module, while the sealed modifier manages the ranges and restricts the lots of subclasses for a sealed class.
What is a sealed interface in Kotlin?
A sealed interact in Kotlin is declared utilizing the sealed modifier and bounds up the feasible count to a finite set internal of the idem file. The software developers use sealed interfaces to present a closed bunch of behaviors or tactics.
What is the use of a sealed interface?
Sealed interfaces in Kotlin serve the goal of defining a bounded bunch of feasible interfaces. Moreover, they let enhanced control and sustainability by limiting the feasibility to a finite count inward of the item file.
What is the difference between sealed interact and Enum Kotlin?
Kotlin uses sealed interfaces and enums to describe a restricted set of variables or parameters. An enumeration cannot be expanded or inherited, whereas a sealed interface can be extended by other interfaces or implemented by classes.
Sealed interfaces define ranges of related types, while enumerations represent a fixed mix of values, such as days of the week, error codes, or dropdown options.
How do I use sealed classes in Kotlin?
To utilize a sealed class in Kotlin, do the following:
- Using the sealed modifier, declare a sealed class.
- In the item file, provide the subclasses of the sealed class.
- Use the sealed class and its subclasses in your code to handle many cases based on the specific subclass, leveraging template matching or when expressions.
Recommends
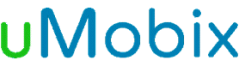
One App to manage all your Agile/
Scrum/Devops Needs
All your work in one place: Task, Project Managment, Bug Tracker, IT Assets, CRM, Docs, Excel, Chat, Goals and more.
Get Free