Kotlin Tutorial for Beginners
Kotlin is a statically typed, cross-platform programming language that runs on the Java Virtual Machine (JVM). It’s an object-oriented language with a concise, expressive, and safe design. Since it’s interoperable with Java, its code can easily fit within existing Java code.
A Simple Kotlin Tutorial on Setting up an Environment
Before starting to read Kotlin tutorial for beginners, you must set up your development environment. Firstly, you must download and install the Kotlin compiler. You can download the compiler from the Kotlin website.
Once the compiler is installed, you must create an Integrated Development Environment (IDE) to write and run your Kotlin code. Popular IDEs supporting Kotlin include IntelliJ IDEA, Android Studio, and Eclipse. In this Kotlin tutorial, we will show an example of creating a kotlin file for Eclipse.
- Download and install the latest version of the Java Development Kit (JDK) from the Oracle website.
- Open Eclipse and click on the “Help” menu.
- Select “Eclipse Marketplace” from the drop-down menu.
- In the Eclipse Marketplace search bar, type “Kotlin” and press Enter.
- You should see the “Kotlin Plugin for Eclipse” in the search results. Click on the “Install” button next to it.
- Follow the installation instructions provided by the plugin installer.
- Once the installation is complete, you can create a new Kotlin file by clicking on “File” menu > “New” > “Kotlin File”.
- In the “New Kotlin File” dialog box, specify the name of the file and the package name.
- Click on “Finish” to create the Kotlin file.
Now you can start writing Kotlin code in your newly created file.
The architecture of Kotlin
Kotlin follows a similar architecture to Java. It has a compiler that turns its code into a bytecode that can run on the Java Virtual Machine (JVM). Alternatively, it can be compiled into native code for specific platforms.
Since Kotlin is object-oriented, everything in it is an object. Moreover, it supports functional programming concepts like lambda expressions and higher-order functions.
Kotlin variable
In Kotlin, variables store data. Typically, one can declare them using the var keyword for variables that allow reassignment. Alternatively, they can use the val keyword for read-only variables. Here’s an example:
var x: Int = 5 val y: String = "Hello, world!"
In this Kotlin example, x is a variable of type Int with an initial value of 5, and y is a read-only variable of type String that has an initial value of “Hello, world!”.
Data Types in Kotlin
Data types supported by Kotlin represent data storable in a variable or an object. It includes numbers, booleans, characters, strings, and arrays. The following table can be helpful for beginners who are just starting to learn Kotlin. It shows the name of each data type and a brief description of what kind of data it can store. Ultimately, it may help understand the basic data types available in the language:
Data Types | Description |
---|---|
Numbers | Stores whole numbers |
Boolean | Stores true or false values |
Char | Stores a single character |
String | Stores a sequence of characters |
Arrays | Stores array data of the same type |
Kotlin Operators
Kotlin supports a wide range of operators, including arithmetic operators, relational operators, and logical operators.
Arithmetic operators:
- Addition: use the “+” operator
- Subtraction: use the “-” operator
- Multiplication: use the “*” operator
- Division: use the “/” operator
- Modulus: use the “%” operator
- Increment: use the “++” operator
- Decrement: use the “–” operator
Relational operators:
- Equal to: use the “==” operator
- Not equal to: use the “!=” operator
- Greater than: use the “>” operator
- Less than: use the “<” operator
- Greater than or equal to: use the “>=” operator
- Less than or equal to: use the “<=” operator
Logical operators:
- Logical AND: use the “&&” operator
- Logical OR: use the “||” operator
- Logical NOT: use the “!” operator
Here are some examples:
var x: Int = 5 var y: Int = 10 var z: Int = x + y // z is now 15. In this example, z is the result of adding x and y var a: Boolean = x < y // a is true because x is less than y var b: Boolean = x > y // b is false because x is not greater than y. val isBothTrue = (x < y) && (y != 0) println("isBothTrue: $isBothTrue") // Output: isBothTrue: true
Kotlin Numbers
In Kotlin, the “Number” is actually an abstract class that serves as a superclass for all the basic numeric types, including Byte, Short, Int, Long, Float, and Double.
- Byte – an 8-bit signed integer (-128 to 127)
- Short – a 16-bit signed integer (-32,768 to 32,767)
- Int – a 32-bit signed integer (-2,147,483,648 to 2,147,483,647)
- Long – a 64-bit signed integer (-9,223,372,036,854,775,808 to 9,223,372,036,854,775,807)
- Float – a 32-bit floating-point number
- Double – a 64-bit floating-point number
All these types inherit from the “Number” class, and they can be used interchangeably in many cases. For example, you can assign an “Int” value to a “Long” variable or a “Float” value to a “Double” variable.
Here’s an example with the Number class:
fun main() { val a: Number = 12.5 // a can hold any numeric value, as it is of type Number val b: Byte = 10 val c: Int = 100 val d: Float = 3.14 f println(a) // output: 12.5 println(b) // output: 10 println(c) // output: 100 println(d) // output: 3.14 // Example of arithmetic operations with different numeric types val sum = b + c // Byte + Int = Int val product = c * d // Int * Float = Float println(sum) // output: 110 println(product) // output: 314.0 }
Kotlin Char
In Kotlin, “Char” is a basic data type that represents a single Unicode character. A character is enclosed in single quotes, like this: ‘a’.
Here’s an example that demonstrates the use of the “Char” data type in Kotlin:
fun main() { val c: Char = 'a' println(c) // output: a val euroSymbol: Char = '\u20AC' println(euroSymbol) // output: € }
In this example, we create two variables of type “Char”. The first variable “c” contains the character ‘a’. The second variable “euroSymbol” contains the Euro symbol, which is represented in Unicode by the hexadecimal value ‘\u20AC’. When we print out the values of these variables, we see that they are printed as single characters.
Note that in Kotlin, a “Char” variable can only hold a single character. If you try to assign a string to a “Char” variable, you will get a compilation error.
Kotlin Boolean
In Kotlin, “Boolean” is a basic data type that represents a truth value. It can only have two values: “true” or “false”.
Here’s an example that demonstrates the use of the “Boolean” data type in Kotlin:
fun main() { val isSunny = true val isRaining = false if (isSunny) { println("It's a sunny day!") } else if (isRaining) { println("It's a rainy day!") } else { println("It's neither sunny nor raining.") } }
In this example, we create two variables “isSunny” and “isRaining” of type “Boolean”. We then use these variables in an “if-else” statement to print out a message based on the current weather conditions. If “isSunny” is true, we print “It’s a sunny day!”. If “isRaining” is true, we print “It’s a rainy day!”. Otherwise, we print “It’s neither sunny nor raining.”.
Note that in Kotlin, “Boolean” variables are often used to represent conditions and control the flow of the program.
What is the Index of an Array in Kotlin?
In Kotlin, an array is a structure of items of the same data type. Each item in the array is assigned a unique index, starting at 0. You can access individual items in an array using their index. Here’s an example:
var arr: IntArray = intArrayOf(1, 2, 3, 4, 5) var item: Int = arr[2] // item is 3
In this example, arr is an array of Int values, and the item is the value at index 2, which is 3.
Kotlin Strings
In Kotlin, strings are represented using the String data type. Strings can be declared using double quotes. Here’s an example:
var str1: String = "Hello, world!"
In this example, str1 string that contain the text “Hello, world!”.
Kotlin Collection
Kotlin supports several collection types, including lists, sets, and maps. Here’s an example of how to declare a list in Kotlin:
var list: List<Int> = listOf(1, 2, 3, 4, 5)
In this example, List is a read-only list of Int values.
Kotlin Functions
Functions are used to group a set of related statements and perform a specific task. In Kotlin, you can declare functions using the fun keyword. Here’s an example:
fun add(x: Int, y: Int): Int { return x + y } var z: Int = add(5, 10) // z is now 15
In this example, add is a function that takes two Int values as arguments and returns their sum.
Exception Handling in Kotlin
Exception handling is an integral part of any programming language. In Kotlin, you can use the try, catch, and finally keywords to handle exceptions. Here’s an example:
try { // code that might throw an exception } catch (e: Exception) { // code to handle the exception } finally { // code that will always run, regardless of whether an exception was thrown }
In this example, the try block contains code that might throw an exception. The catch block is responsible for any handling exceptions you may throw. Code in the finally block always executes, regardless of whether you issue an exception.
Kotlin Null Safety
One of the main features of Kotlin is its built-in null safety features. The language helps prevent null pointer exceptions by design, eliminating one of the common sources of bugs in programming. Kotlin achieves this by using a system that distinguishes between nullable and non-nullable types.
In Kotlin, you can declare a null safety by specifying whether a variable or parameter can be nullable or not.
To declare a nullable variable, you can use the ‘?’ operator after the type:
val myNullableString: String? = null
In this example, myNullableString is a nullable String variable that is initialized to null.
To declare a non-nullable variable, you simply leave off the ‘?’ operator:
val myNonNullString: String = "Hello, world!"
In this example, myNonNullString is a non-nullable String variable that is initialized to the string “Hello, world!”.
Here’s an example of how to use Kotlin Null Safety:
fun calculateStringLength(str: String ? ): Int { if (str != null) { return str.length } else { return 0 } }
The following example defines a calculateStringLength function that takes a nullable string as an argument. The ? after the String type indicates that the string may be null.
Inside the function, you must first check whether str is null. If it’s not null, you return the string length using the length property. If it is null, you return 0 instead.
Arguably, this code is the exemplar of safe null handling because it checks for null before attempting to access the length property of the string. Without null safety, attempting to access a property or method of a null object would result in a null pointer exception.
Another way to use Kotlin Null Safety is with the safe call operator (?.) and the Elvis operator (?:):
fun printFirstLetter(str: String ? ) { val firstLetter = str ? .get(0) ? : "N/A" println("The first letter is $firstLetter") }
In this example, you define a printFirstLetter function that takes a nullable string as an argument. The safe call operator then accesses the first character of the string using the get() method. If the string is null, the safe call operator returns null and the Elvis operator provides a default value of “N/A”. Consequently, you can print the first letter to the console.
Kotlin Extensions
Kotlin extensions are a feature of the Kotlin programming language that allows developers to add new functionality to existing classes, without the need to modify the source code of those classes.
Kotlin extensions are defined as functions that are defined outside of the class but are still associated with that class. Extensions can be defined for any class.
To define an extension function, you use the “fun” keyword followed by the name of the function, the name of the class that the function is extending, and the function parameters.
Here is an example of an extension function that adds a “reverse” method to the String class:
fun String.reverse(): String { return this.reversed() }
In this example, the extension function is defined for the String class using the “receiver type” syntax. The function itself simply calls the built-in “reversed()” method of the String class to reverse the string and return the result.
Objects in Kotlin
In Kotlin, objects are unique instances of classes. They can be created using the object keyword, allowing the developer to use them throughout the app. Such an object doesn’t require much coding and opts for instantiating only in the first access.
Here’s an example:
object MySingleton { fun doSomething() { println("I'm doing something!") } }
In this example, MySingleton is an object that has a single function called doSomething. It allows you to call the doSomething function from anywhere in the app using the following prompt:
MySingleton.doSomething()
Kotlin Inheritance
Inheritance is a tool of object-oriented programming that enables creation of new classes based on existing classes. In Kotlin, you can use the open keyword to indicate that a class can be inherited from. Here’s an example:
open class Animal { open fun makeSound() { println("The animal makes a sound") } } class Dog: Animal() { override fun makeSound() { println("The dog barks") } } var myDog: Animal = Dog() myDog.makeSound() // prints "The dog barks"
In this example, Animal is a base class with a single function called makeSound. The Dog class is a derived class that inherits from Animal. The Dog class overrides the makeSound function to make the dog bark.
Abstract Class in Kotlin
An abstract class is a type that cannot be instantiated. Abstract classes are used as base classes for other classes. In Kotlin, you can use the abstract keyword to indicate that a class is abstract. Here’s an example Kotlin code:
abstract class Animal { abstract fun makeSound() } class Dog: Animal() { override fun makeSound() { println("The dog barks") } } var myDog: Animal = Dog() myDog.makeSound() // prints "The dog barks"
In this example, Animal is an abstract class with a single abstract function called makeSound. The Dog class is derived from Animal and overrides the makeSound function to make the dog bark.
History of Kotlin
JetBrains is a software development company based in Russia that began working on Kotlin in 2010. The product’s first public beta launched in 2011, addressing some of the limitations of Java. Some of the main issues that Kotlin addresses regarding Java include null safety, type inference, and extension functions.
Why should you use Kotlin?
You might want to use Kotlin for your programming projects for several reasons. Some of the main reasons include the following:
- Easy to learn: Kotlin is a modern, easy-to-learn language that is concise and expressive.
- Interoperable with Java: It is interoperable with Java, so you can easily integrate Kotlin code with existing Java code.
- Safe: Kotlin has built-in null safety features that help prevent errors and bugs.
- Concise: Kotlin is concise, meaning you can write less code to accomplish the same tasks.
- Versatile: It is suitable for various computing tasks, from mobile app development to web development.
Kotlin Features
Kotlin has several features that make it an attractive programming language for developers. Some of the main features of Kotlin include:
- Null safety: Kotlin has built-in null safety features that help prevent errors and bugs.
- Extension functions: The language allows adding new functions to existing classes without modifying the original class.
- Type interface: It can automatically infer the type of a variable based on its value.
- Lambda expressions: Kotlin supports lambda expressions, which allow you to write more concise and expressive code.
- Coroutines: Kotlin has built-in support for coroutines, lightweight threads that allow you to write asynchronous code more naturally.
Here’s a simple Kotlin programming language tutorial.
The Present & Future of Kotlin
Even though Kotlin is a relatively new programming language, it has already gained much popularity. Companies like Pinterest, Uber, and Trello actively use it, while Android employs it as its official language for app development. In this regard, it’s safe to say that the future of Kotlin looks bright. JetBrains, the company that developed Kotlin, is committed to supporting and improving the language. Moreover, the open-source community shows increasing support, further fueling the language’s growth.
Kotlin is also being used by Google to develop new features for the Android operating system, which means that its use is likely to continue to grow in the coming years.
In conclusion, Kotlin is a versatile and easy-to-learn programming language that is perfect for beginners. With this beginner’s guide to Kotlin, you’ll grasp the basics of programming and become a skilled developer. Ultimately, Kotlin is the perfect language to help you achieve your goals.
FAQ
Is Kotlin easy to learn for beginners?
Yes, many believe Kotlin to be a generally easy language for beginners. It has a clean and concise syntax, which makes it easy to read and write. Moreover, it’s interoperable with Java, which allows developers with Java experience to switch to Kotlin without a hassle. While some basic programming concepts are helpful, even beginners with no prior experience can start learning them and become proficient.
Is Kotlin better than Python?
It’s difficult to say whether Kotlin is “better” than Python, as both languages have their strengths and weaknesses depending on the specific use case. The first is generally a better choice for building robust and scalable applications running on the JVM. On the other hand, Python is excellent for scripting, data analysis, and machine learning and has a more expressive and concise syntax. Ultimately, the choice depends on the specific requirements of the project.
Is Kotlin more complex than Java?
No, Kotlin is generally easier than Java because of its concise and expressive syntax. Moreover, it’s more readable and intuitive and eliminates many common pitfalls and boilerplate code that developers often encounter when using Java.
Moreover, the Kotlin syntax is generally more modern and expressive, making it easier to read and understand.
Is Kotlin easier to learn than Python?
Kotlin and Python are relatively easy to learn but have different strengths and weaknesses. JetBrains’ language has a more structured and static typing system, while Python has a simpler and more expressive syntax. Ultimately, the difficulty of learning depends on the individual developer’s background and experience and the project’s specific requirements.
Can I use Kotlin to create Android apps?
Yes, Kotlin is a popular language for Android app development. As already mentioned, Google officially supports Kotlin as an Android development language.
Suppose you want to create an Android app using Kotlin. Here’s how a “Hello, World!” sample would look like:
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val helloTextView = findViewById<TextView>(R.id.text_view)
helloTextView.text = “Hello, World!”
}
}
While the sample code is primitive, it demonstrates the merits of creating Android apps using Kotlin.
Are there any resources to help me learn Kotlin?
Yes, there are many resources available to help you learn Kotlin. The list includes online courses, tutorials, books, and more.
Can I create games using Kotlin?
Yes, you can use Kotlin to build games. The language is versatile and is suitable for many different types of programs, including games. Besides, it’s perfect for creating apps on Android.
Recommends
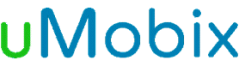
One App to manage all your Agile/
Scrum/Devops Needs
All your work in one place: Task, Project Managment, Bug Tracker, IT Assets, CRM, Docs, Excel, Chat, Goals and more.
Get Free